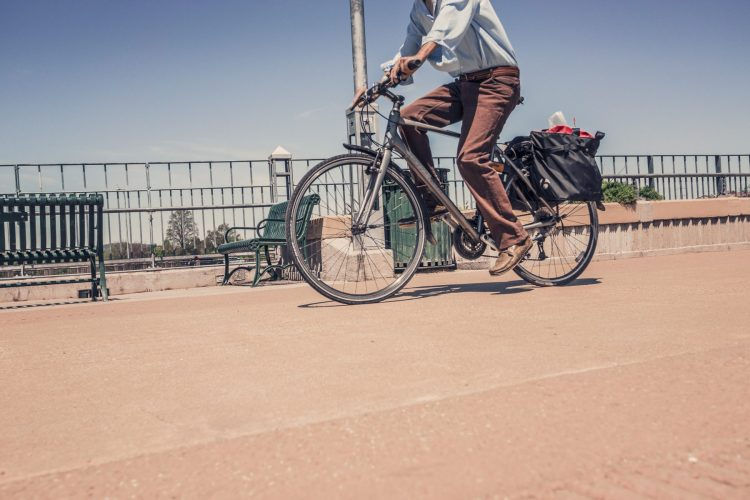
Hieronder vind je de code die behoort bij het artikel SpecFlow Extensies voor Dummies
Feature File
Feature: Table Transformations
In order to have convenient datatypes
As a SpecFlow user
I want to transform tables into dictionaries or datatables
Scenario: Transform a vertical table into a dictionary
Given I have the following table:
| Movie | Rating |
| The Matrix | 8.5 |
| It | 8.1 |
When I transform this table into a dictionary
Then the dictionary should have a key "The Matrix" with a value "8.5"
And the dictionary should have a key "It" with a value "8.1"
Scenario: Transform a table into a datatable
Given I have the following table:
| Movie | Rating | Director |
| The Matrix | 8.5 | The Wachowskis |
| It | 8.1 | Tommy Lee Wallace |
When I transform this table into a datatable
Then the datatable should have the following record:
| Column | Value |
| Movie | The Matrix |
| Rating | 8.5 |
| Director | The Wachowskis |
And the datatable should have the following record:
| Column | Value |
| Movie | It |
| Rating | 8.1 |
| Director | Tommy Lee Wallace |
Step Definiton:
using System.Collections.Generic;
using System.Linq;
using TechTalk.SpecFlow;
using SpecFlowExamples.Extensions;
using Microsoft.VisualStudio.TestTools.UnitTesting;
using System.Data;
namespace SpecFlowExamples.Steps
{
[Binding]
public class TableSteps
{
[Given(@"I have the following table:")]
public void GivenIHaveTheFollowingTable(Table table)
{
ScenarioContext.Current.Add("table", table);
}
[When(@"I transform this table into a dictionary")]
public void WhenITransformThisTableIntoADictionary()
{
var table = (Table)ScenarioContext.Current["table"];
var dictionary = table.ToDictionary();
ScenarioContext.Current.Add("dictionary", dictionary);
}
[Then(@"the dictionary should have a key ""(.*)"" with a value ""(.*)""")]
public void ThenTheDictionaryShouldHaveAKeyWithAValue(string key, string value)
{
var dictionary = (Dictionary<string, string>)ScenarioContext.Current["dictionary"];
Assert.IsTrue(dictionary.ContainsKey(key), "The dictionary contains key " + key);
Assert.AreEqual(value, dictionary[key]);
}
[When(@"I transform this table into a datatable")]
public void WhenITransformThisTableIntoADatatable()
{
var table = (Table)ScenarioContext.Current["table"];
var dataTable = table.ToDataTable();
ScenarioContext.Current.Add("datatable", dataTable);
}
[Then(@"the datatable should have the following record:")]
public void ThenTheDatatableShouldHaveTheFollowingRecord(Table table)
{
var dataTable = (DataTable)ScenarioContext.Current["datatable"];
var expectedRecord = table.ToDictionary();
foreach (DataRow row in dataTable.Rows)
{
var matchingRowFound = true;
foreach (var keyValuePair in expectedRecord)
{
var column = keyValuePair.Key;
var value = keyValuePair.Value;
if (!value.Equals(row[column]))
{
matchingRowFound = false;
break;
}
}
if (matchingRowFound)
return;
}
Assert.Fail("The table does not contain the record.");
}
}
}
SpecFlow.Table Extension:
using System.Collections.Generic;
using System.Linq;
using TechTalk.SpecFlow;
using SpecFlowExamples.Extensions;
using Microsoft.VisualStudio.TestTools.UnitTesting;
using System.Data;
namespace SpecFlowExamples.Steps
{
[Binding]
public class TableSteps
{
[Given(@"I have the following table:")]
public void GivenIHaveTheFollowingTable(Table table)
{
ScenarioContext.Current.Add("table", table);
}
[When(@"I transform this table into a dictionary")]
public void WhenITransformThisTableIntoADictionary()
{
var table = (Table)ScenarioContext.Current["table"];
var dictionary = table.ToDictionary();
ScenarioContext.Current.Add("dictionary", dictionary);
}
[Then(@"the dictionary should have a key ""(.*)"" with a value ""(.*)""")]
public void ThenTheDictionaryShouldHaveAKeyWithAValue(string key, string value)
{
var dictionary = (Dictionary<string, string>)ScenarioContext.Current["dictionary"];
Assert.IsTrue(dictionary.ContainsKey(key), "The dictionary contains key " + key);
Assert.AreEqual(value, dictionary[key]);
}
[When(@"I transform this table into a datatable")]
public void WhenITransformThisTableIntoADatatable()
{
var table = (Table)ScenarioContext.Current["table"];
var dataTable = table.ToDataTable();
ScenarioContext.Current.Add("datatable", dataTable);
}
[Then(@"the datatable should have the following record:")]
public void ThenTheDatatableShouldHaveTheFollowingRecord(Table table)
{
var dataTable = (DataTable)ScenarioContext.Current["datatable"];
var expectedRecord = table.ToDictionary();
foreach (DataRow row in dataTable.Rows)
{
var matchingRowFound = true;
foreach (var keyValuePair in expectedRecord)
{
var column = keyValuePair.Key;
var value = keyValuePair.Value;
if (!value.Equals(row[column]))
{
matchingRowFound = false;
break;
}
}
if (matchingRowFound)
return;
}
Assert.Fail("The table does not contain the record.");
}
}
}
Comentarios